The Power of Common Table Expressions (CTEs) in SQL

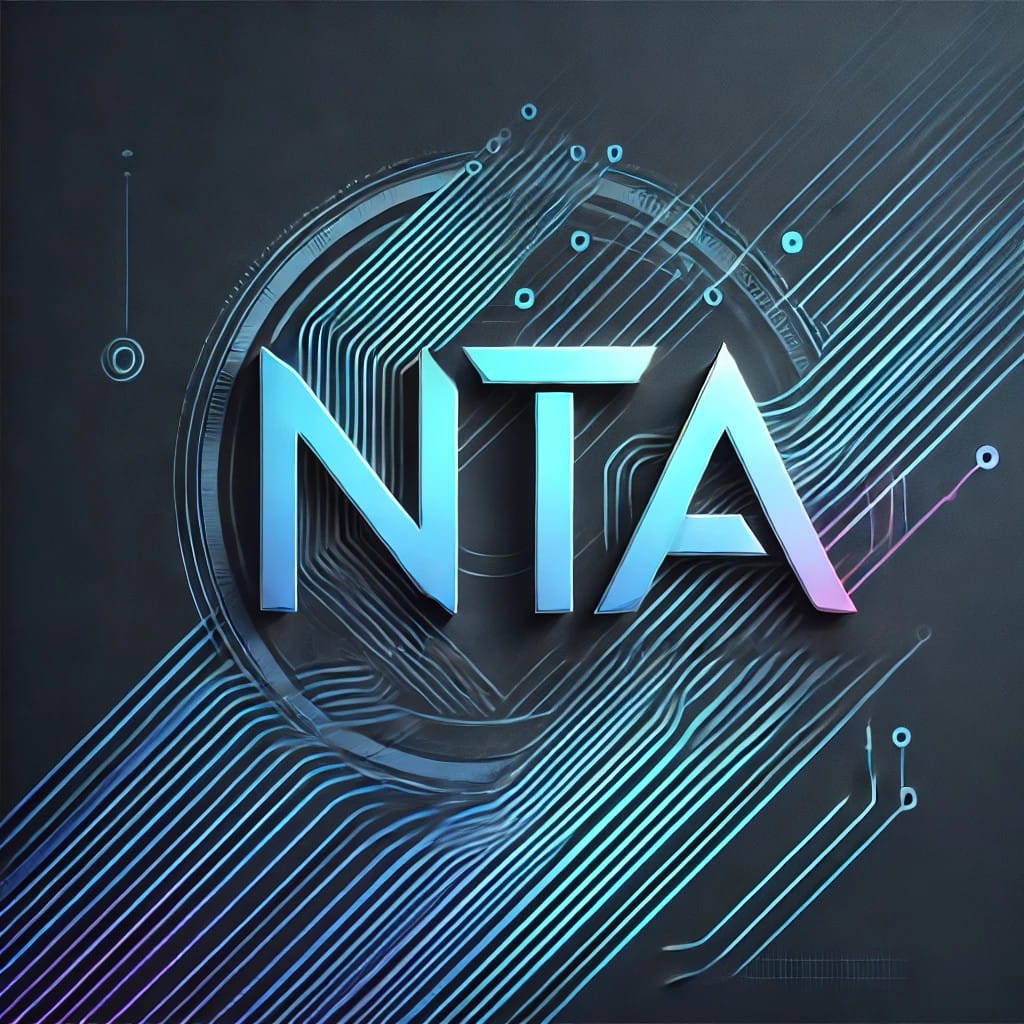
Neural Tech
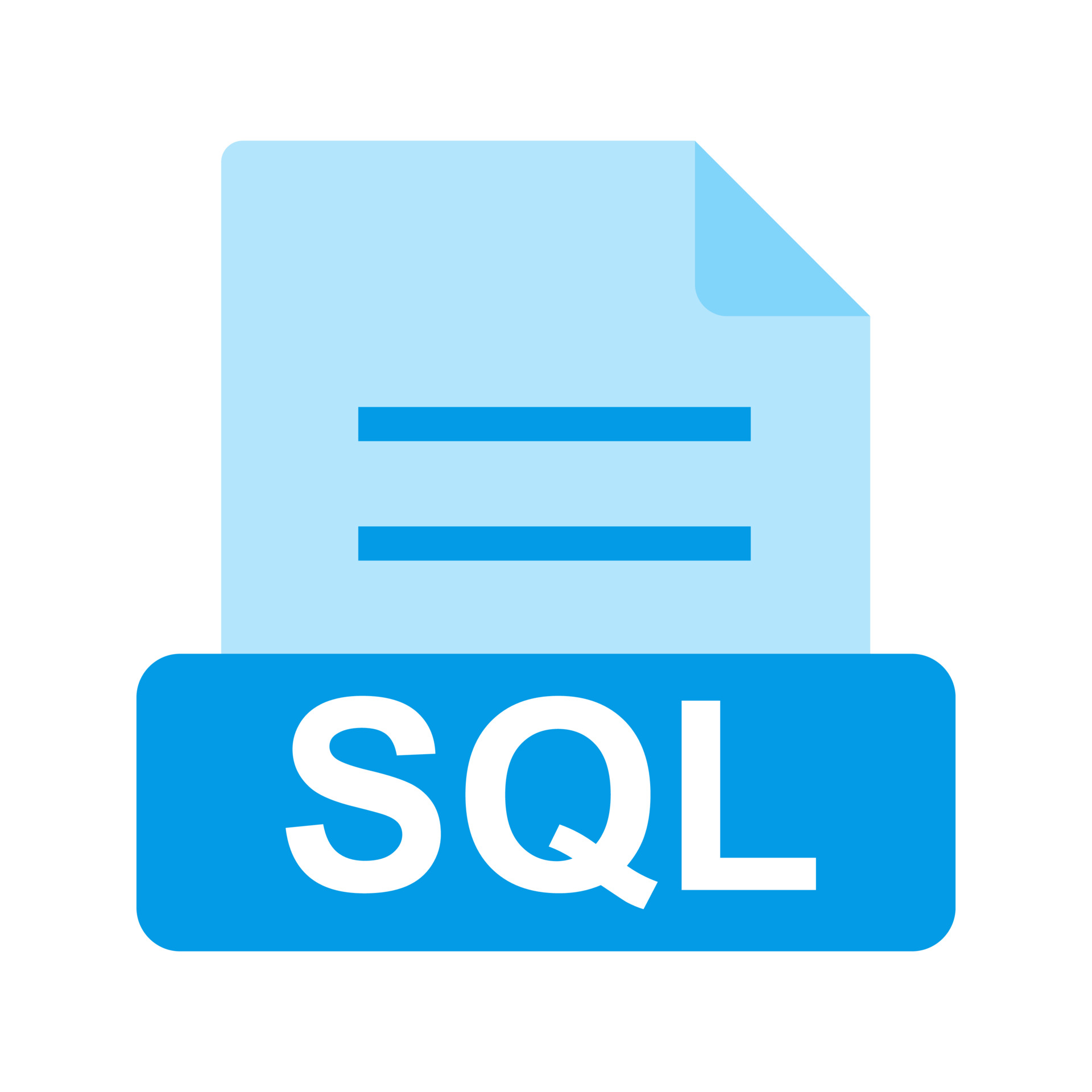
SQL
Ever written an SQL query that turned into a tangled mess of subqueries? You know, the kind where debugging feels like unraveling a mystery novel?
Ever written an SQL query that turned into a tangled mess of subqueries? You know, the kind where debugging feels like unraveling a mystery novel?
- That’s where Common Table Expressions (CTEs) step in. Think of them as temporary tables within your query—except they don’t clutter your database, and they make your SQL way more readable. Let’s break it down.
What Exactly is a CTE?
- A Common Table Expression (CTE) is like a shortcut. Instead of repeating complex logic in multiple places, you define it once and use it throughout your query.
- Here’s the basic idea:
WITH HighSalaryEmployees AS ( SELECT department, employee_id FROM employees WHERE salary > 50000 ) SELECT department, COUNT(*) FROM HighSalaryEmployees GROUP BY department;
- Let’s compare this to the old-school subquery approach:
SELECT department, COUNT(*) FROM ( SELECT department, employee_id FROM employees WHERE salary > 50000 ) AS subquery GROUP BY department;
- The second query works, but it’s harder to read. Now imagine adding more logic—things can get ugly fast. With a CTE, you break the query into logical steps, making it much easier to follow.
Why Should You Care About CTEs?
- Code Readability: No more long, confusing queries.
- Better Query Performance: Optimized execution plans in many cases.
- Reusability: Define it once, use it multiple times.
- Recursive Queries: Perfect for handling hierarchical data like org charts.
Real-World Example: Employee Hierarchy
- Ever had to work with manager-employee relationships in a database? Recursion makes it easy!
- Here’s how to fetch an employee hierarchy using a recursive CTE:
WITH EmployeeHierarchy AS ( SELECT employee_id, manager_id, employee_name, 1 AS hierarchy_level FROM employees WHERE manager_id IS NULL -- Start from the top-level manager UNION ALL SELECT e.employee_id, e.manager_id, e.employee_name, eh.hierarchy_level + 1 FROM employees e INNER JOIN EmployeeHierarchy eh ON e.manager_id = eh.employee_id ) SELECT * FROM EmployeeHierarchy;
- This recursive CTE keeps fetching employees until the entire hierarchy is built. No loops, no complicated joins, just pure SQL magic!