SQL Query Optimization
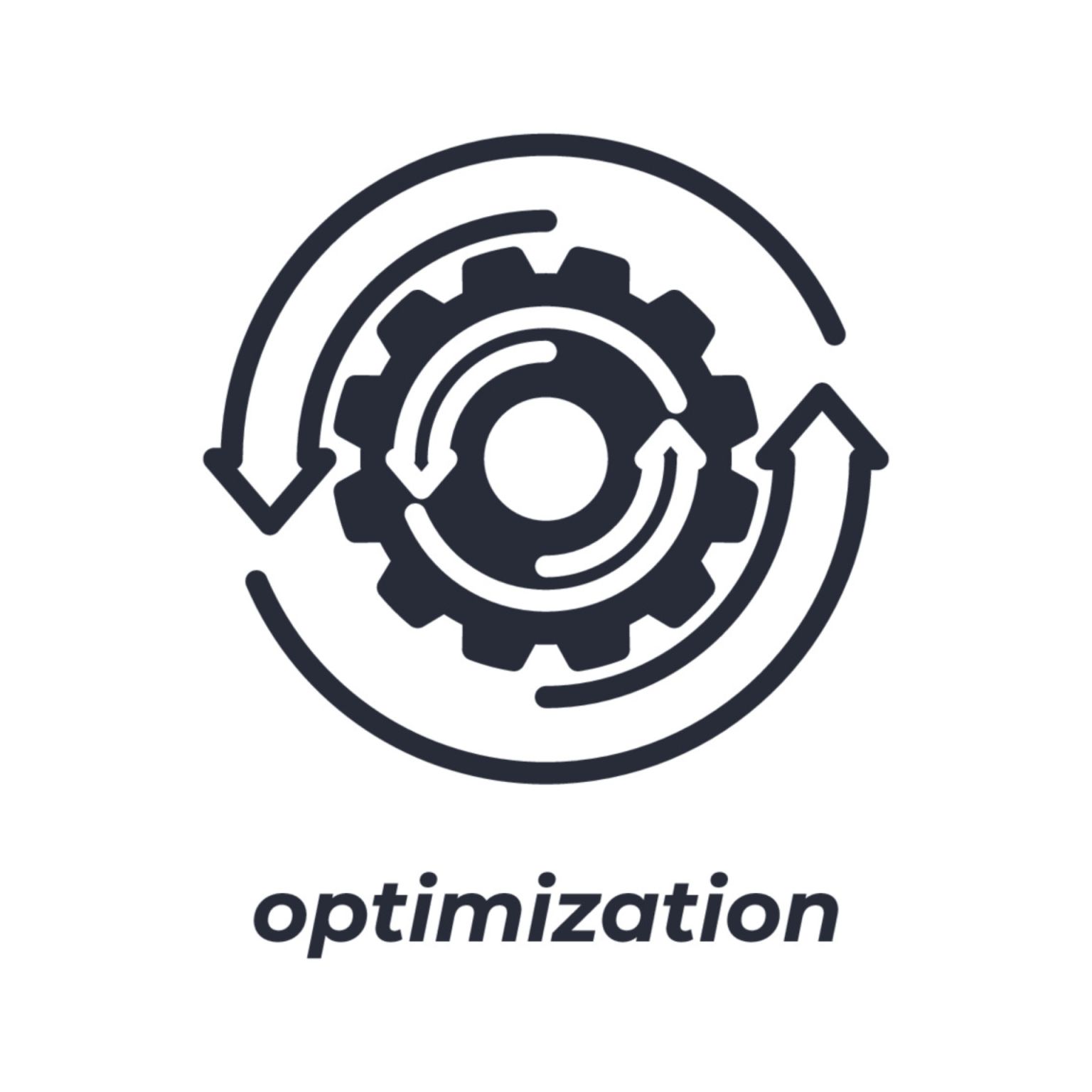
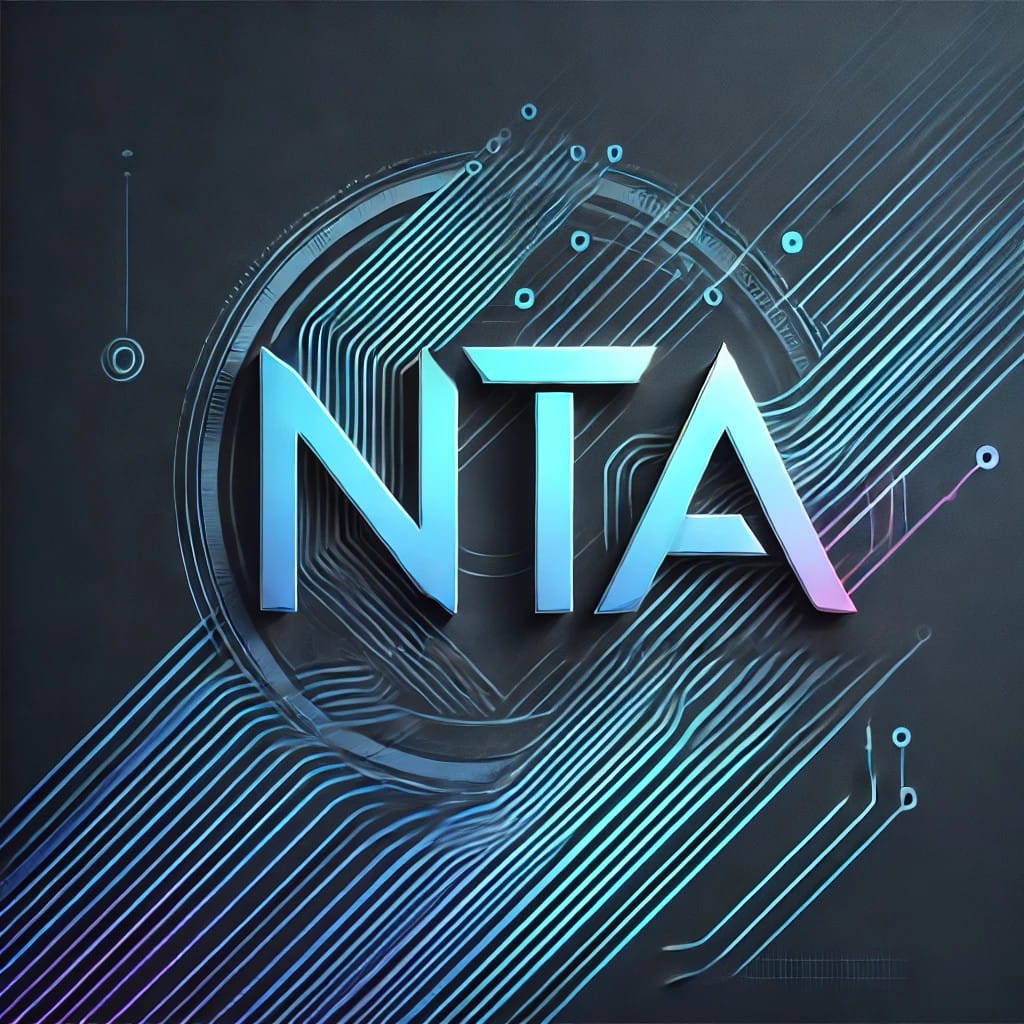
Neural Tech
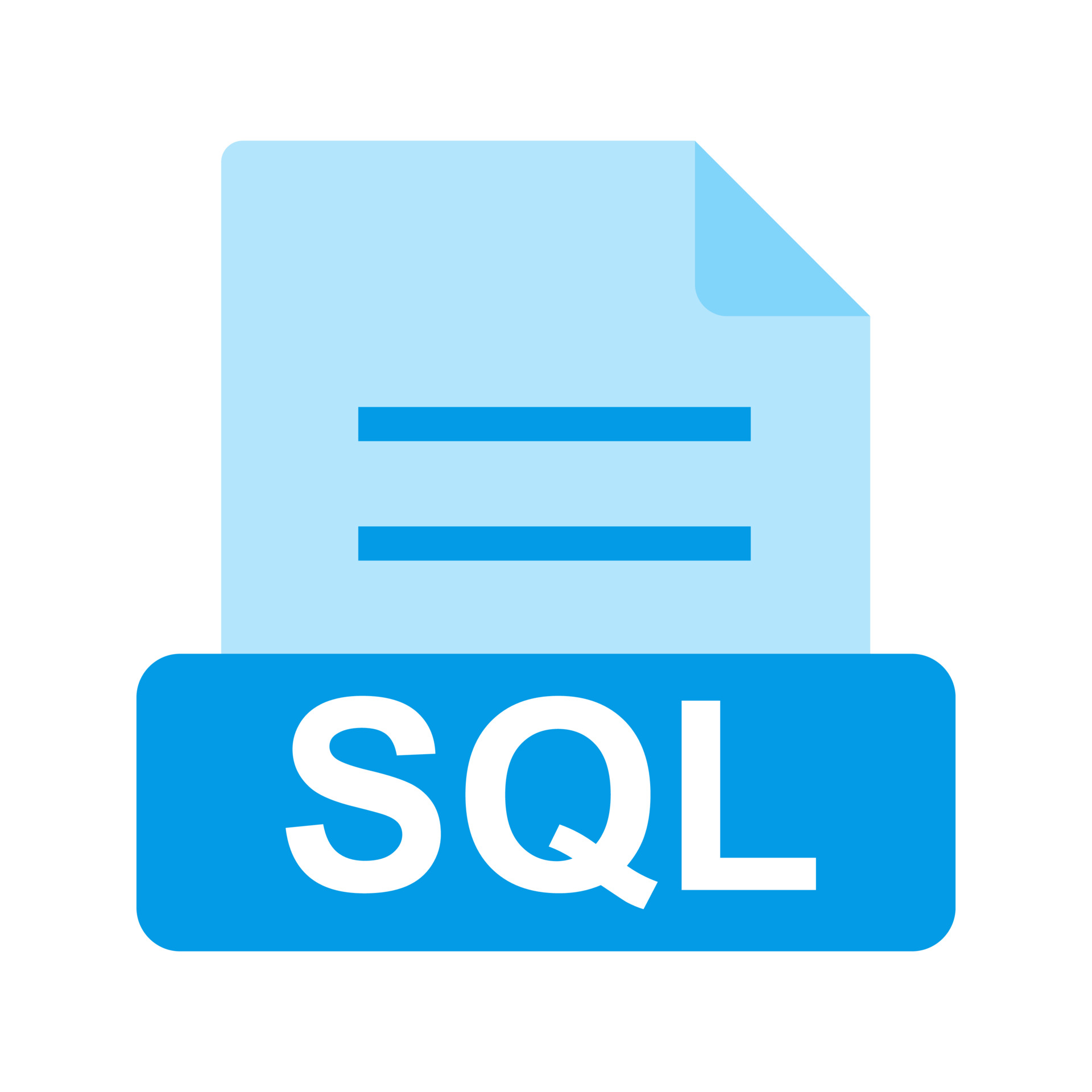
SQL
Ever written an SQL query that takes forever to run? Most slow queries can be optimized with just a few smart tweaks. Let’s break it down.
Ever written an SQL query that takes forever to run? Most slow queries can be optimized with just a few smart tweaks. Let’s break it down.
-
Stop Using (SELECT *), Only Fetch What You Need:
-
When you use (SELECT *), you are retrieving every column, even the ones you don’t need. This increases data transfer time and slows things down.
-
-
Add Indexes, Make Searching Lightning Fast:
-
Indexes act like a table of contents in a book. Instead of scanning every row, the database jumps straight to the relevant data.
-
Without Index: Your database does a full table scan, which is slow.
-
With Index: It quickly finds rows using a B-tree structure.
-
-
Avoid Unnecessary JOINs, They Slow Everything Down:
-
JOINs are powerful but can be expensive in terms of performance. If your query is slow, check if you really need all those JOINs.
-
Instead of multiple JOINs, consider:
-
Denormalizing data, Store frequently accessed information in one table instead of spreading it across many.
-
Using Indexed JOINs, Ensure JOIN keys have indexes for faster lookups.
-
Use EXISTS Instead of IN for Subqueries:
-
EXISTS is often faster than IN, especially in large datasets. Why?
-
IN retrieves all matching values first, then filters.
-
EXISTS stops searching as soon as it finds a match.
-
-
Partition Large Tables, Speed Up Query Processing:
-
If your table has millions of rows, scanning everything is slow. Partitioning splits data into smaller, manageable chunks, making queries much faster.
-
-
Use WHERE Instead of HAVING (When Possible):
-
The HAVING clause filters data AFTER aggregation, while WHERE filters before aggregation.
-
Reduces the number of rows before grouping, making queries much faster.
-